Native HTML lazyload in React
React does not come with built-in lazy loading for images, but it can be easily implemented using various techniques and third-party libraries.
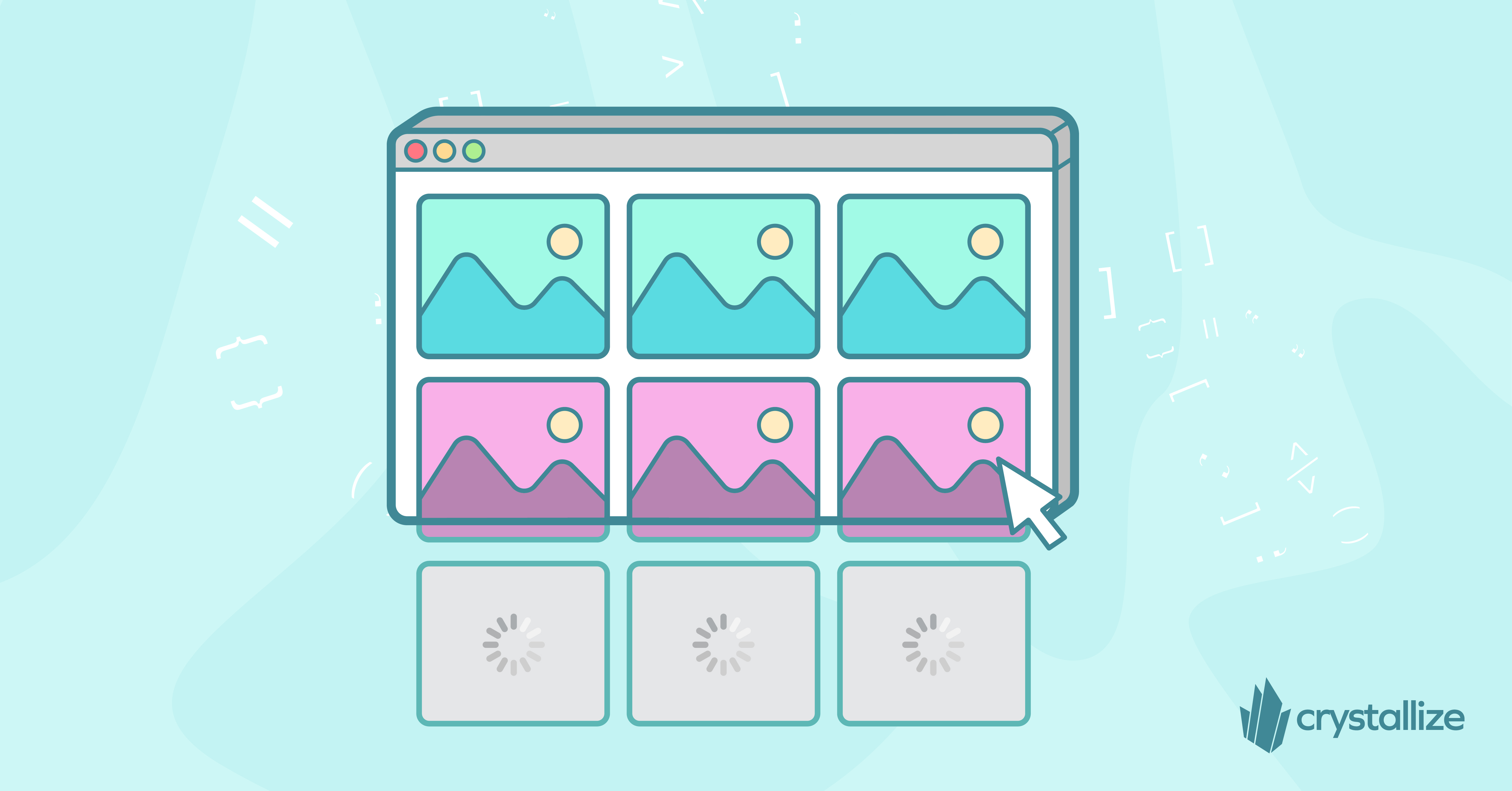
The users of your React eCommerce love a speedy experience. One key aspect of ensuring great speed is to load the data the users need when they need it. Lazy loading is a must-have tool in your developer tool belt since it enables you to do just that, elegantly loading the images from your Product Information Management just when the users want to see them.
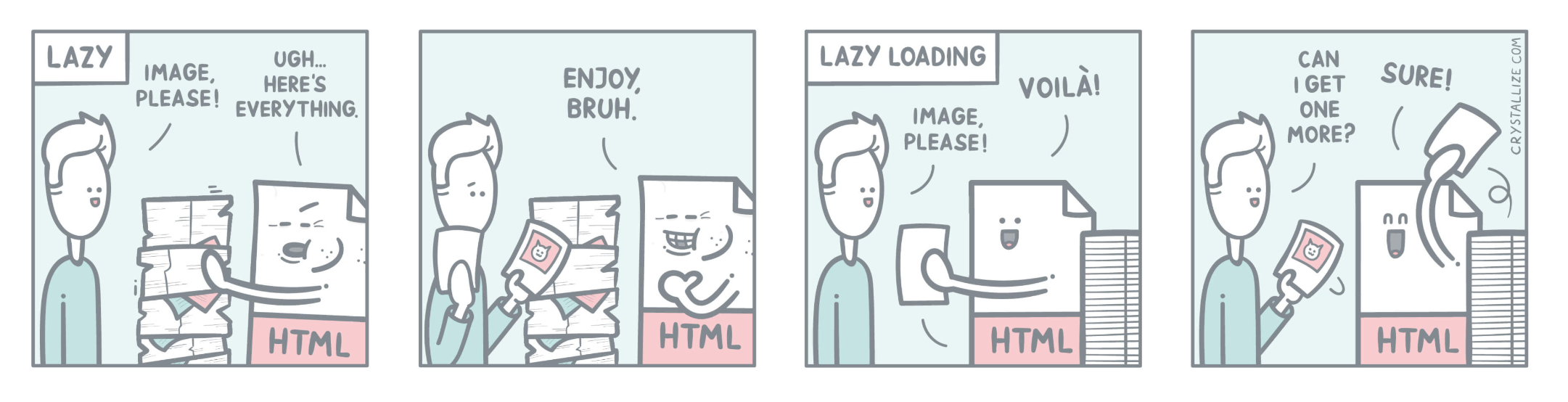
The Problem
Embedding an image on an HTML page is straightforward:
<img src=”image.webp” />
If you want to show multiple images, you simply do multiple image tags:
<img src=”image1.webp” />
<img src=”image2.webp” />
<img src=”image3.webp” />
<img src=”image4.webp” />
Now, what is the problem with this approach? It might not be apparent by looking at those lines of HTML, and it has to do with how web browsers render that HTML on the screen and how quickly it is able to do that.
With every image, there is a download cost. The browser picks up all the image tags on the page and downloads them when the page loads. This is the core of the problem. The page-load is the most critical time during a user session. A lot of things are happening at the same time, and all assets are fighting for bandwidth and CPU time.
The more images, the more bandwidth and CPU time they take, delaying the loading of other assets on the page, like videos, fonts, and scripts, essentially blocking major parts of the user journey for all between sub-seconds to minutes.
This might not be a big problem for the code above, but what if you had 400 images instead of 4? In that case, you have a real problem on your hands.
Native HTML lazy loading
For images and iframes, the simplest method to implement lazy loading is by using the native loading
attribute, which is supported in modern browsers. This method does not require any additional libraries or complex configurations.
Here’s how you do it:
<img src=”image.webp” loading=”lazy” />
The image will be loaded when the browser sees it fit, typically when the user has scrolled near the image. Fantastic! In the latest version of Chrome, an image is now downloaded if it’s 1250px near the viewport edge, giving the browser enough time to download the image before the user has scrolled to it.
The downside of this is that since the browser has not downloaded the image, it will take up no space in the layout, rendered as width=0, height=0. To compensate for that, you have to provide the height and width values:
<img src=”image.webp” loading=”lazy” width="100" height="100" />
This will ensure that the space of the image is reserved, and you’ll not get any layout shifts when the browser decides to download the image.
Using React Lazy for Components
For lazy loading React components, you can use React's built-in React.lazy function alongside Suspense. This method is ideal for splitting your app into smaller chunks and loading them on demand.
Using Third-Party Libraries
For more sophisticated or specific lazy loading scenarios (like images, components, or even routes), you might consider using a third-party library. React-lazyload is one such library that provides versatile lazy loading capabilities.
Installation:
bashCopy code
npm install react-lazyload --save
Usage. Wrap your components or images with LazyLoad
components.
jsxCopy code
import LazyLoad from 'react-lazyload';
<LazyLoad height={200} offset={100}>
<img src="image-url.jpg" alt="description" />
</LazyLoad>
In this example, height
specifies the height of the placeholder before the actual content loads, and offset
determines how far from the viewport you want to start loading your content.
Speedy Loading with Crystallize react-image Module
Once the browser determines that your image is ready to be downloaded, this must happen fast. Make sure to use a Content Delivery Network (CDN) or a platform that offers that as part of its service.
You get this out-of-the-box for all your media assets, i.e., images and videos, if you're using Crystallize.
Oh, and if you’re using our react-image module, you pass the loading attribute the same way as you would for a regular img tag:
import { Image } from '@crystallize/react-image';
export const LazyImage = (crystallizeImage) => <Image {...crystallizeImage} loading="lazy" />
Conclusion
Most of the images on any webpage should have loading=lazy by default. Only critical, above-the-fold images should be loaded simultaneously, and the browser will handle the heavy lifting for you.
For further details and how to use this feature, check out Browser Level Image Lazy Loading article.
BTW, CDN support for your media is just one of the features you get with Crystallize. Schedule a 1-on-1 demo so we can show you what else you get out of the box with Crystallize and how we can help you future-proof and grow your business.