GraphQL Typescript Generators
GraphQL is a query language that optimizes performance by returning only the requested data, while TypeScript is a statically-typed language that helps catch errors during development. Together they can create robust and efficient web applications.
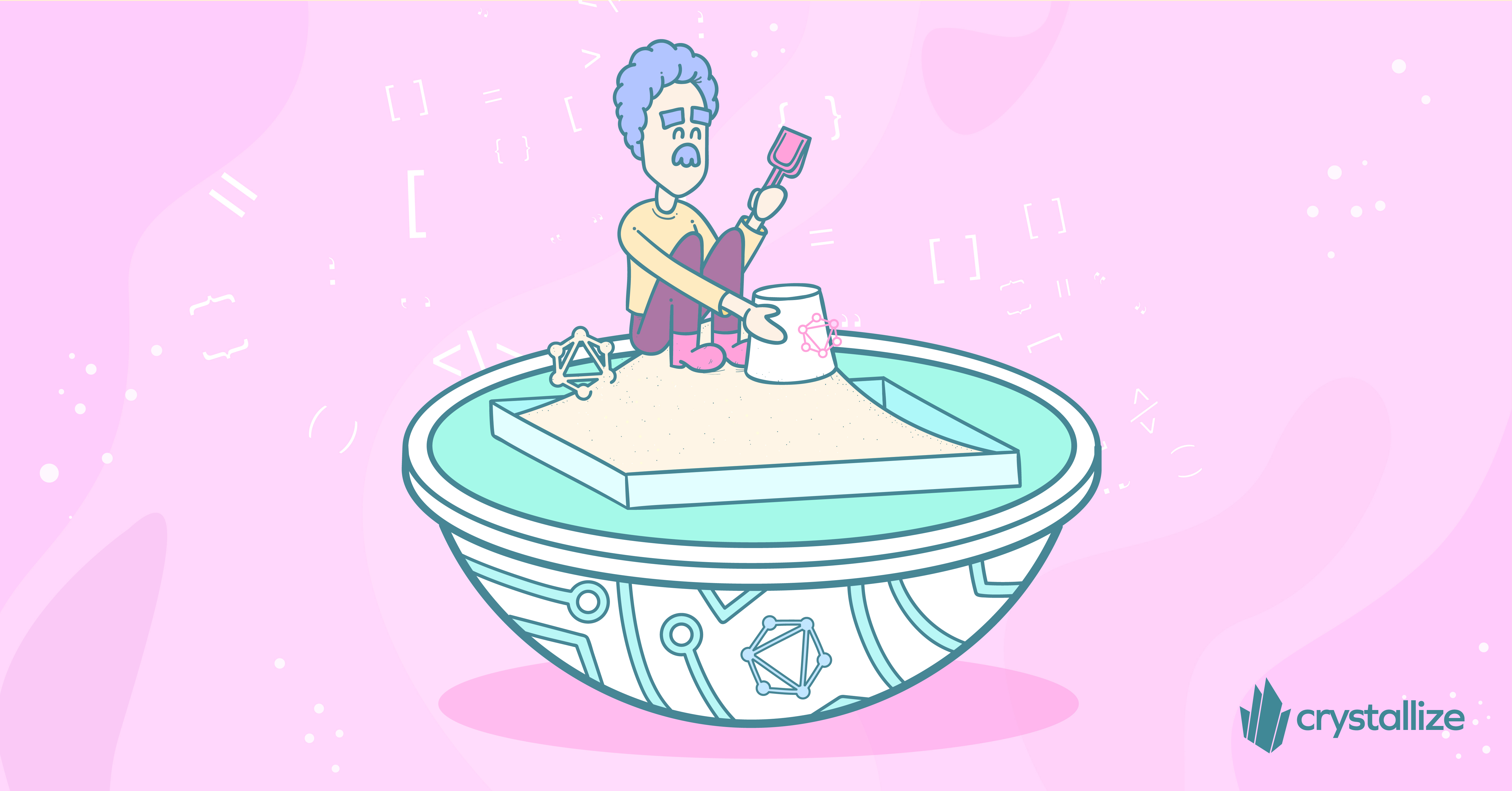
GraphQL is a popular query language used for building APIs. With GraphQL, developers can specify the data requirements of their application, and the server returns only the requested data. This approach helps optimize performance and reduce the amount of data transmitted between the client and server. On the other hand, TypeScript is a popular statically-typed language that helps catch errors during development.
Using GraphQL with TypeScript has become increasingly popular due to its benefits in type safety, which ensures catching errors in the development phase.
However, creating the necessary types for GraphQL queries and mutations can be a tedious and error-prone process. GraphQL TypeScript generators can automate this process and generate the necessary types, making development faster and more efficient.
In this blog post, we will take a closer look at GraphQL TypeScript generators, how they work, and why they are useful.
What Are GraphQL TypeScript Generators?
GraphQL TypeScript generators are tools that automatically generate TypeScript types from GraphQL schemas. These tools can generate types for queries, mutations, and subscriptions.
By using these types, developers can ensure that the data they are accessing from the server is correctly typed and errors are caught during development.
There are several GraphQL TypeScript generators you can choose from, the most popular ones being:
These tools can be used with any GraphQL API, regardless of the programming language used to implement the API.
How Do GraphQL TypeScript Generators Work?
GraphQL TypeScript generators work by analyzing the GraphQL schema and generating TypeScript types based on the schema. The generators use the GraphQL schema to infer the types of fields and arguments in the queries and mutations. Once the types are inferred, the generators create TypeScript types that can then be used in client-side code.
The generators typically use templates to generate the TypeScript code, which allows developers to customize the generated code to match their specific use case. The templates can be written in a variety of languages, including TypeScript, JavaScript, and even GraphQL SDL.
Why are GraphQL TypeScript generators useful?
There are several benefits of using GraphQL TypeScript generators. Let’s take a look at the most prominent ones.
Improved Productivity
By automating the generation of TypeScript types, developers can save a significant amount of time and effort during development. This allows developers to focus on building the core features of their application rather than worrying about the type safety of their code.
Improved Type Safety
Using TypeScript with GraphQL can significantly improve the type safety of an application. By generating TypeScript types from the GraphQL schema, developers can ensure that the data they are accessing from the server is correctly typed and errors are caught during development.
Reduced Errors
Using TypeScript with GraphQL can help catch errors early in the development process. This can reduce the number of bugs that make it to production, improving the overall quality of the code.
Simplified Maintenance
As the application evolves, the GraphQL schema may change. By using a GraphQL TypeScript generator, developers can easily update the TypeScript types to reflect the changes in the schema. This can help simplify maintenance and reduce the risk of errors.
Example🔎
Alright! Now that we have an overview of what GraphQL TypeScript generators are let’s take a look at an example below. The API we will be using for this example is Crystallize’s Catalogue API.
Step 1: Set Up the Project
First, we need to create a new project and install the required packages. To create a new project, open your terminal and type the following command:
mkdir crystallize-api && cd crystallize-api
Next, we need to initialize our project with npm or yarn. For this tutorial, we will be using npm but feel free to use whatever you prefer.
npm init -y
Once we have initialized our project, we need to install the following packages:
npm install graphql graphql-codegen graphql-request @graphql-codegen/cli
- graphql: the official GraphQL library for JavaScript.
- graphql-codegen: tool that generates TypeScript types from a GraphQL schema.
- graphql-request: library for making GraphQL requests.
- @graphql-codegen/cli: command-line interface for graphql-codegen.
Step 2: Set Up the GraphQL Endpoint
Next, we need to set up our GraphQL endpoint. For this tutorial, we will be using Crystallize's Catalogue API. The endpoint for the API is:
<https://api.crystallize.com/{your-tenant-name}/catalogue>
Please make sure to add your tenant identifier there.
Step 3: Configure graphql-codegen
Next, we need to configure graphql-codegen to generate types for our Catalogue API. Create a new file called codegen.yml in your project's root directory and add the following code:
overwrite: true
schema: <https://api.crystallize.com/frntr/catalogue>
documents: ./src/**/*.graphql
generates: { "./src/gql/": { preset: "client" } }
This configuration file tells graphql-codegen to generate TypeScript types for the Catalogue API and output the generated code to the ./src/gql folder.
Step 4: Add a Query
Now that we have our configuration file setup, it’s time to add a GraphQL query so we can generate types for it. The example query we are using for this tutorial is a simple hello-world query:
query HelloCrystallize{
catalogue(language: "en", path: "/shop") {
name
children {
name
path
}
}
}
Step 5: Generate the Types
Finally, it is now time to generate the types! To make it easier, let’s add a script to our package.json file:
"generate": "graphql-codegen --config codegen.yml"
Now that we have configured graphql-codegen, we can generate the TypeScript types by running the following command in the terminal:
npm run generate
This command tells graphql-codegen to read the configuration from the codegen.yml file and generate TypeScript types for the Catalogue API. Once graphql-codegen has finished generating the TypeScript types, you should see newly generated files in the **src/gql** folder.
Conclusion
GraphQL TypeScript generators can significantly improve the development process when working with GraphQL APIs. By automating the generation of TypeScript types, developers can improve productivity, type safety, and reduce errors. With several tools available, developers can choose the tool that best fits their needs and start reaping the benefits of GraphQL TypeScript generators in their applications.
Should you use a GraphQL TypeScript generator for your project? Why not!
It is a great way to ensure type safety. There is one thing that needs to be kept in mind here, though, this intrinsically couples your API and business logic. If you’d rather have the API and the business logic decoupled, then something like data transfer object (DTO) approach is worth looking into.