Web Rendering Explained: SSR, CSR & SSG for High-Performance Sites
Server-side Rendering, Static Site Generation, Client-side Rendering. CSR with Prerendering, Progressive Hydration, Partial Pre rendering, etc. The differences, trade-offs, and similarities between most common web rendering solutions and why you should care about them as a developer.
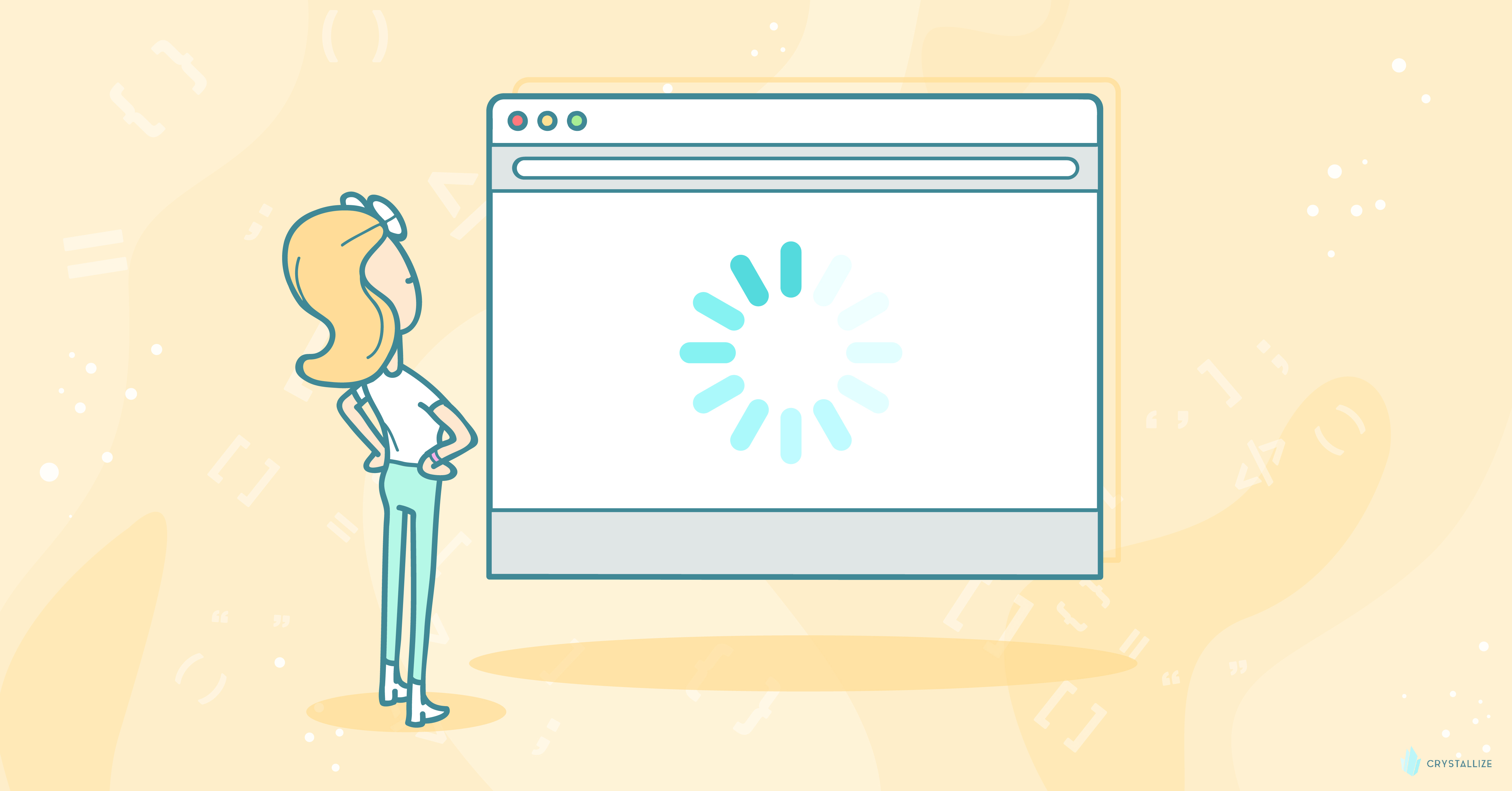
Whenever you write about frameworks, you end up with 50% of the article trying to explain concepts around rendering on the web. So, the topic deserves its own post, this one.
Before we get started, we need some background and definitions. What is rendering on the web? To answer that question, we'll take a stroll down history lane.
More than thirty years ago, the web started as a collection of static documents built for scientists to share their findings and ideas. Over the following decades, the web became more dynamic, and technologies like PHP, MySQL, and others enabled developers to build more dynamic functionalities.
Since then, JS frameworks and a class of static site generators have been introduced, rendering has become complex, and different models are suitable for different use cases. This article attempts to make sense of all this complexity. If we succeed, you will be empowered to determine which methodologies are best suited for your next web project.
Considerations
When choosing a technology for a project, here are some considerations (familiarity and preferences aside):
- SEO: You can choose the right technologies accordingly, depending on whether SEO is important for your project.
- How dynamic is this project, is that an e-commerce (highly dynamic) or a blog (mostly static)?
- Performance: performance is always a consideration when choosing technologies.
- Hosting: Where do you host your e-commerce frontend? Different rendering method also affects what kind of hosting platform you can use and how expensive your hosting cost may be,
There are other technical considerations that you should consider, such as TTFB, TTI, FCP, etc. However, those are beyond the scope of this article.
(BTW, most tech considerations/best practices are covered in our best practices for web development with modern frameworks blog post.)
Rendering Options
One of the main goals, alongside explaining the rendering approaches and their pros and cons, is to clear up the acronym's confusion about rendering on the Web. So, while it might seem that the following subtitles are not in order, there is one which is part of a bigger picture.
Server-side Rendering (SSR)
Server-side rendering is when the server generates the full HTML document in response to the browser's navigation request. This usually involves a template engine with data fetched from the database. Here's what happens from start to end:
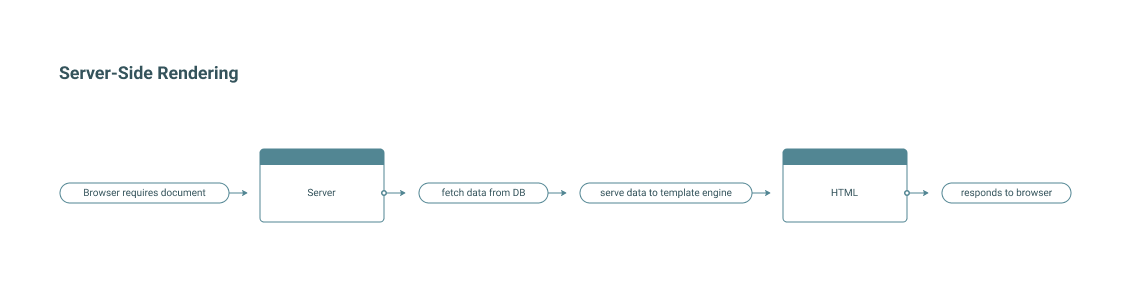
SSR may be slower than other methods when there are many steps between the request and response. However, the content is highly dynamic because the server fetches data from the data source at every request. However, SSR is increasingly used with static data. This is how Next.js or Astro work: server-side rendered, no javascript involved, and the HTML is shipped to the browser. You may have heard of React Server Components, the React Framework, but executed server side.
The drawback of SSR is that Javascript must be shipped at some point to make the application dynamic, and different frameworks have different methods to achieve that.
Use cases: eCommerce websites, content-heavy sites, and platforms requiring good SEO.
Legacy frameworks: Ruby on Rails, PHP Laravel.
Modern framework: Next.js, Astro, React Router v7 (Remix), and SvelteKit are named Backend for Frontend Framework (BFF). They resolve the JavaScript shipping issue by combining SSR with highly dynamic UI, shipping only the amount of JavaScript needed for interactivity, making them the best choice in 2025. They are SSR + progressive enhancement. See Hydration and Progressive Hydration and SSR with Hydration.
Static Rendering (with Static Site Generation - SSG)
Static Rendering is when the server builds all pages into HTML documents. This is called a "build step". The end result of the build step is a set of HTML documents that can be deployed to a server or CDN, ready to "serve."
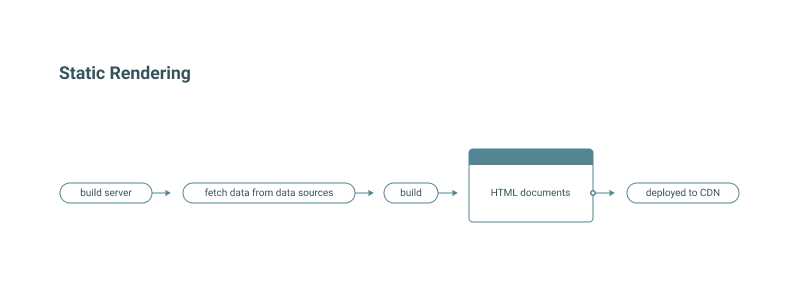
The main characteristic of this method is static. That's both the pros and cons of this method.
Because all pages are static, they produce a super-fast load time. They are readily available and easily scaled globally. However, they are also very inflexible and non-dynamic. Because of this, the SSG approach is mostly popular for blogs, simple landing pages, and brochure websites.
Use cases: Blogs, marketing sites, and documentation platforms.
Frameworks: Astro, Gatsby (remember this one), Next.js (Static Export mode).
Client-side Rendering (CSR)
Client-side rendering occurs when the server (ideally the CDN) delivers a static application shell to the browser and allows the JavaScript code to handle the rendering on the client. An application that uses CSR is typically referred to as a single-page application (SPA), as it consists of just one page, and routes are managed by the application instead of the server.
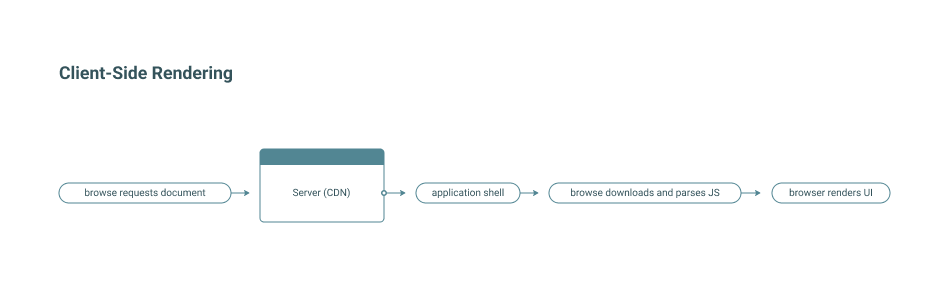
This is usually the default rendering method when you use client-side JavaScript frameworks. It's usually simpler to get started as you don't need to use a meta-framework (framework on top of a framework) or further optimization. However, it comes with some significant drawbacks.
The first issue is around load time. While the document size may be smaller because it's only the application shell, the browser may take a while to parse the JavaScript code before users can view and interact with the content that needs to be fetched after the first load. Techniques like lazy loading and code splitting are important when using CSR.
The second issue concerns SEO. Since the server only delivers an application shell, the browser must rely on JavaScript to fully load and function the page. Although Google crawlers can parse JavaScript and render your web pages, this topic is still quite controversial. Many experiments and lessons learned from switching between CSR and SSR indicate that SSR is superior in SEO.
Given these drawbacks, CSR only makes sense if your web projects don't require SEO. Its most popular use cases are web applications (typically back-office or desktop applications), SaaS dashboards, etc. Dig into effective strategies for optimizing React eCommerce SPAs for SEO here.
Use cases: Single-page applications (SPAs), dashboards, and internal tools.
Example frameworks: React, Vue, Angular, Svelte.
Hydration and Progressive Hydration
Hydration is the process of attaching JavaScript functionality to server-rendered HTML, making the page interactive in the browser. When a page is rendered server-side or pre-generated, it delivers static HTML to the user.
Hydration enables dynamic interactions, such as clicking buttons or updating forms, by reactivating JavaScript on the client side. While this makes applications interactive, traditional hydration often loads all JavaScript upfront, which can delay the page from becoming fully usable.
Progressive Hydration refines this concept by prioritizing critical content and deferring JavaScript activation for non-essential interactive elements. For example, visible content like a product title is hydrated first, while secondary elements like carousels or filters are hydrated later as needed.
This approach improves perceived performance by minimizing initial JavaScript payloads and focusing resources where they matter most, enhancing speed and user experience. Progressive Hydration is especially effective for performance-critical applications like eCommerce and content-heavy platforms.
Progressive hydration requires determining which parts of the page should be hydrated first to optimize perceived performance. This often requires careful prioritization of critical, user-facing content. Managing JavaScript bundles for progressive hydration involves breaking them into smaller chunks, loading them in sequence, and ensuring dependencies are resolved without blocking the main thread.
SSR with Hydration
The three solutions mentioned above (SSR, SSG, and CSR) are the foundations of web rendering. Of course, each has pros and cons. To combat the cons, developers have attempted to combine those techniques.
SSR with Hydration is a mesh of SSR and CSR. The idea is to build dynamic pages that are good with SEO while highly interactive with a JS framework.

This technique would be great if you could take advantage of the benefits of SSR and CSR, with SSR being good for SEO and CSR being good for interactivity. However, to achieve that, you do have to pay for both SSR and CSR.
SSR with Hydration delivers fast initial load times and excellent SEO by serving pre-rendered HTML but introduces performance trade-offs due to hydration overhead, where the browser reinitializes interactivity with large JavaScript bundles, increasing Time-to-Interactive (TTI).
Streaming SSR
Streaming SSR is an advanced server-side rendering technique that progressively sends HTML in chunks to the browser rather than waiting for the entire page to be generated. This reduces time-to-first-byte (TTFB), allowing users to see and interact with initial content faster.
The browser can start rendering the partial HTML immediately, improving perceived performanceâespecially on slower connections. After the initial chunk arrives, the server sends subsequent HTML pieces until the full page is rendered. Developers often combine streaming SSR with client-side hydration to ensure the dynamic parts of the application remain interactive.
This is called Partial Pre Rendering in Next.js, for instance. The page is SSR rendered and might be static (cached), and the dynamic part comes with streaming using Suspense boundaries. This might become the default way.
Use cases: E-commerce product pages with numerous recommended items or user-customizable options and real-time dashboards that need to display partial data immediately while further data is streamed.
Frameworks: Next.js, Remix Run, Nuxt 3, SvelteKit.
CSR with Prerendering
CSR with Prerendering combines CSR and static generation. The idea is to pre-build all HTML pages with a static site generator to make the site fast, SEO-friendly, and highly interactive after JS kicks in.
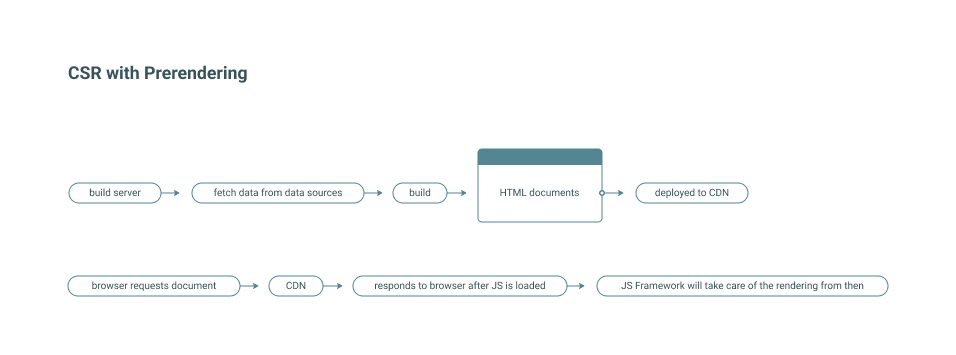
This technique is one of the best ways to deliver a lightning-fast, interactive website. Its main drawback is the same as that of the static rendering technique. Since you must build all pages at build time, dealing with highly dynamic content is more challenging. It may not be suitable for projects with user-generated SEO requirements (blogging platform, forum, etc.).
Incremental Static Regeneration (ISR)
Incremental Static Regeneration (ISR) is a rendering approach that combines the speed of static site generation (SSG) with the flexibility of server-side rendering (SSR). With ISR, static pages can be re-generated at runtime, ensuring they stay up-to-date without requiring a full site rebuild.
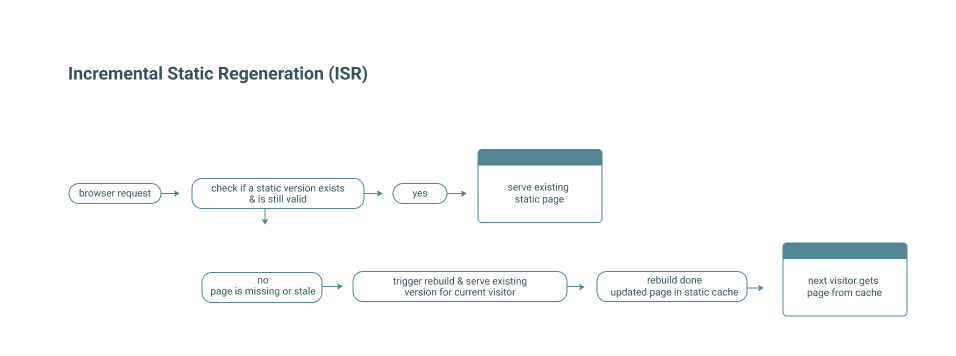
When a user visits a page that needs updating, the server generates a fresh version in the background and caches it for subsequent visitors. This enables developers to serve pre-rendered content quickly while ensuring that frequently changing data, like product inventory or news updates, remains current.
With Incremental Static Regeneration (ISR), latency issues can arise during the cache refresh cycle. Users might receive outdated content until the server completes the regeneration process. Real-time updates are particularly challenging when dealing with highly dynamic data, as the delay between a page request and its regeneration can impact user trust and experience.
Use cases: eCommerce sites with changing inventory or news platforms.
Frameworks: Next.js.
Edge Side Rendering (ESR)
With Edge-side Rendering (ESR), content is rendered and served at edge servers, which are geographically distributed closer to users. By rendering at the edge, ESR reduces latency and improves page load times, as content doesnât need to travel from a central server. It can handle dynamic content efficiently, making it ideal for applications like eCommerce or personalized user dashboards where speed is critical.
With ESR, rendering can occur dynamically or through pre-caching strategies, ensuring up-to-date content is served without compromising performance. This approach combines the benefits of server-side rendering (SSR) with the performance advantages of Content Delivery Networks (CDNs).
The main challenge with Edge-Side Rendering (ESR) is distributing and synchronizing dynamic data across geographically distributed edge servers. Updates must propagate efficiently without significant delays.
Use cases: High-performance global applications.
Frameworks: Next.js with Vercel or Cloudflare Workers.
Distributed Persistent Rendering (DPR)
Distributed Persistent Rendering (DPR) is a modern rendering approach that combines static site generation (SSG) with on-demand dynamic rendering optimized for scalability and performance. Unlike traditional SSG, which pre-builds all pages during deployment, DPR generates pages dynamically when they are first requested and caches the result persistently for subsequent visits.
The "distributed" aspect refers to using edge networks or globally distributed servers to handle rendering, ensuring low latency and fast delivery regardless of user location. By caching the rendered pages, DPR avoids redundant rendering while keeping content fresh and responsive to user needs. Netlify was one of the first supporters of DPR workflows.
Use case: Highly efficient for large-scale websites with a vast number of pages, such as eCommerce stores.Â
Frameworks: Next.js with Netlify.
Comparison
Each rendering approach serves specific needs, and choosing the right one depends on factors like performance, SEO requirements, interactivity, and content dynamism. We've created a simple comparison table explaining the differences. However, keep in mind that many modern frameworks support multiple rendering modes to accommodate diverse project requirements, so assess the project needs and frontend possibilities before choosing the right rendering approach.
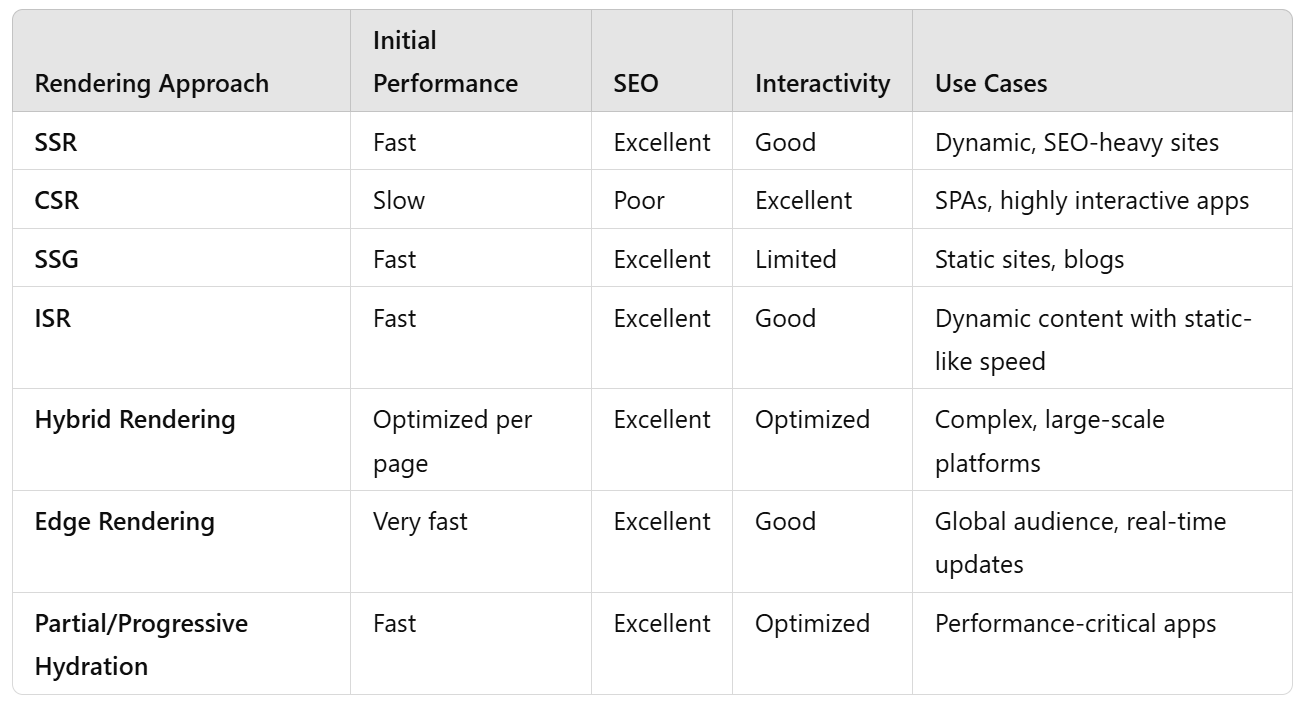
Examples: Rails, Laravel, Phoenix, Django
These are all server-side web frameworks. These technologies mostly use SSR as their main rendering method. Optimization techniques and libraries enable them to be used with a client-side JS framework, turning them into SSR with Hydration.
Examples: Most Client-Side JS Frameworks
Most client-side JS frameworks such as Angular, React, Svelte, Vue default to CSR as its rendering method. There are meta-frameworks on top that enable further rendering options.
Some example meta-frameworks:
- Angular: Angular Universal
- React: Next.js, Gatsby, Remix
- Svelte: SvelteKit
- Vue: Nuxt, Vuepress
Examples: Versatile Next.js
Using Next.js, developers can use SSG, SSR with Hydration, and CSR with Prerendering, depending on the use case. Next.js is like the Swiss army knife of a web framework, and that's why it's a useful tool to have in every developer's toolbelt. That must be one of the reasons Next.js has exploded in popularity over the past few years (source: State of JS 2024).
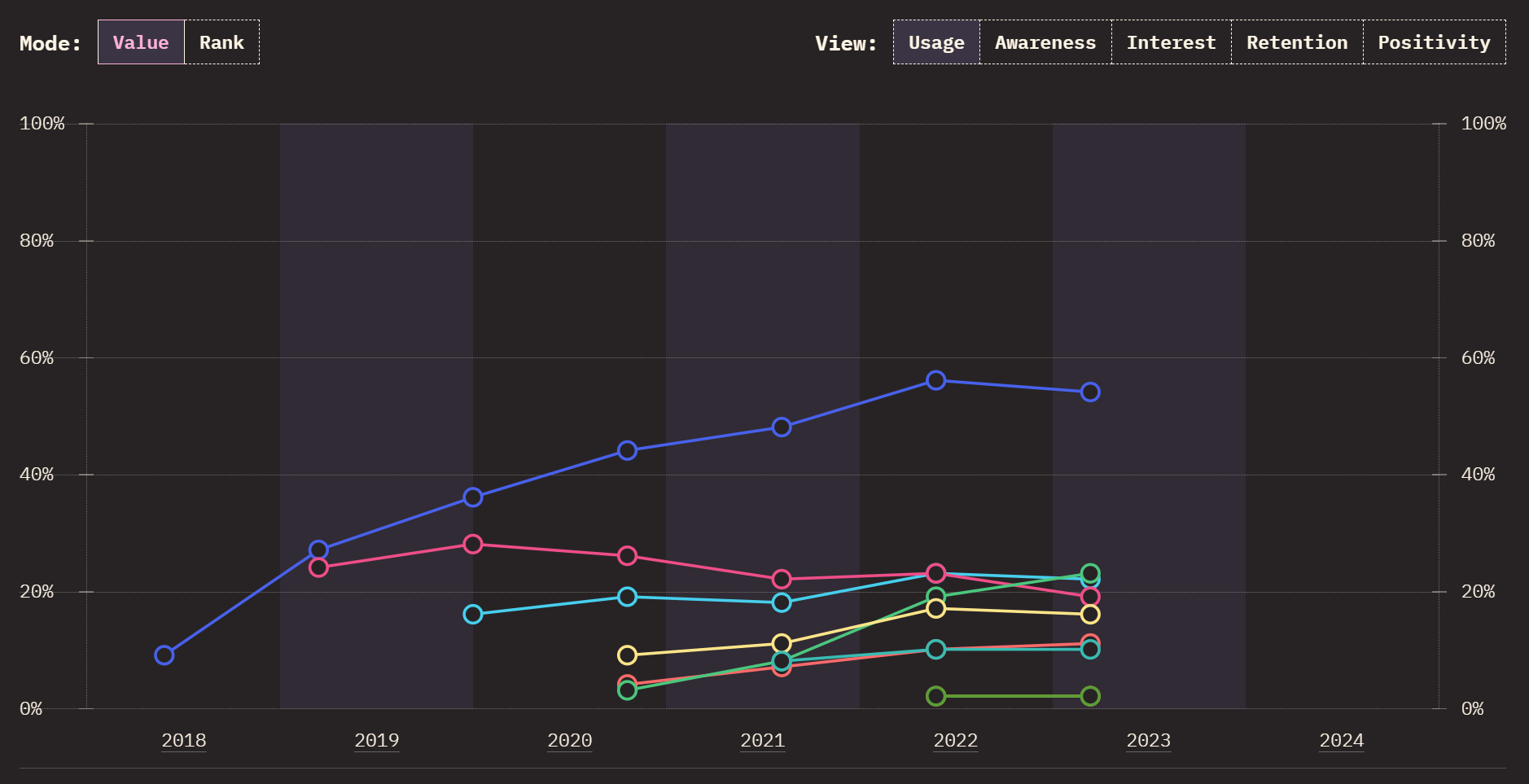
What Now?
Hopefully, this article has explained the difference between using different web technologies. Which one you should go with boils down to your project and its needs.
Thinking of switching to an eCommerce platform + frontend framework that helps rather than hinders your website's performance but not sure of the approach you should take?
Donât worry. We got your back!
Set up a personal 1-on-1 demo today, and letâs see what solution best fits your use case. Or, why not SIGN UP for FREE and start building.
Further Reading
Here are some additional great reads if you're choosing a front-end technology for your web projects:
Populating the page: how browsers work - https://developer.mozilla.org/en-US/docs/Web/Performance/How_browsers_workÂ
Rendering on the Web by Google ChromiumDev team - https://web.dev/articles/rendering-on-the-webÂ
Website rendering strategies Google Search Central video - https://www.youtube.com/watch?v=XMYrqhdJFxU
Follow the Rabbitđ°
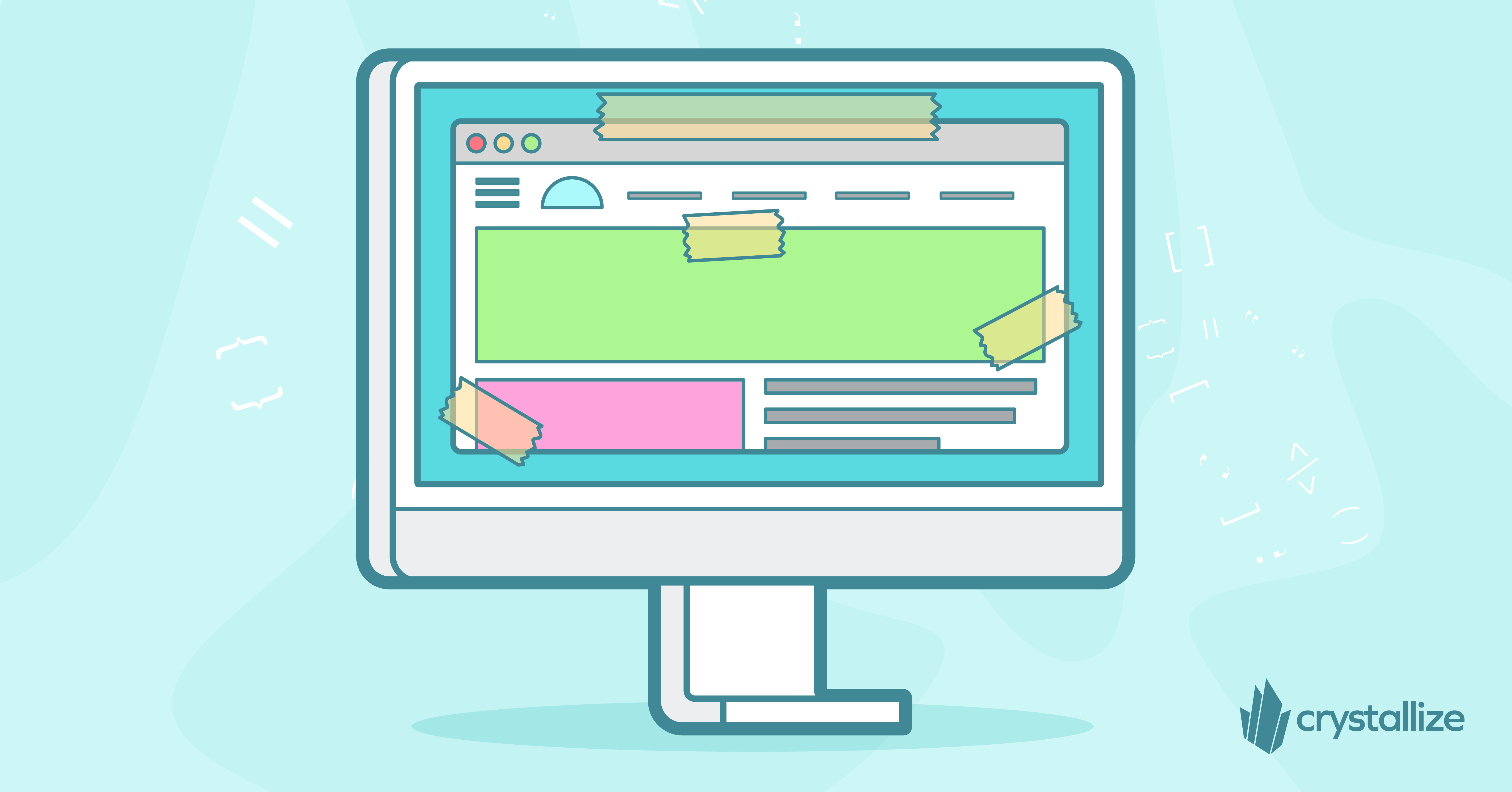
What Is a Static Site Today?
There are numerous approaches to developing a web application that is performant. Going the static site way is just one of them. Pause here. What do we/I mean when we/I say static sites?
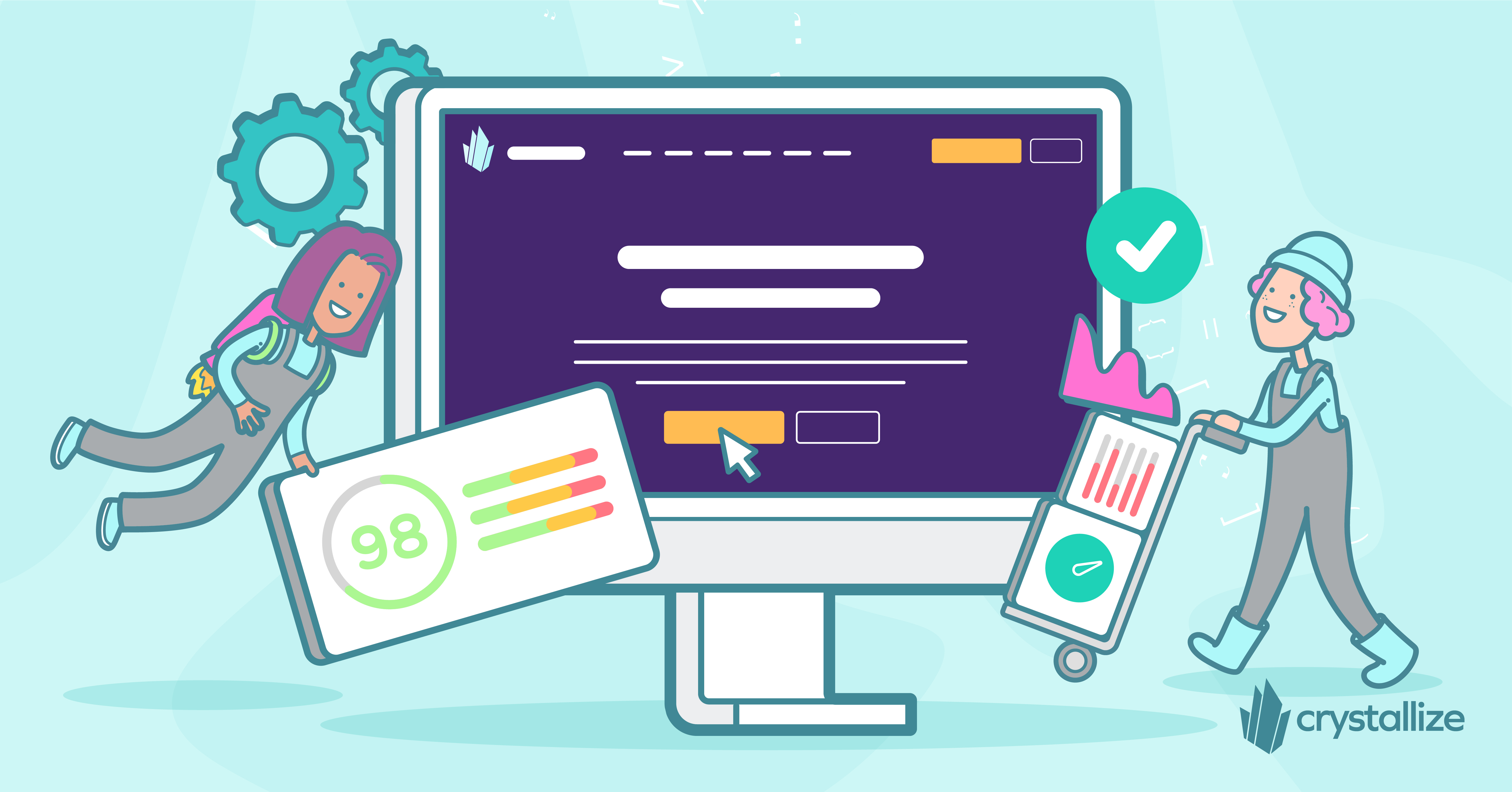
Optimizing eCommerce Success: Best Practices for Web Development with Modern Frameworks
Unlock the potential of your online store with modern frameworks and eCommerce web development best practices for building a fast, user-friendly, and high-converting site.